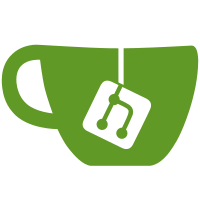
3 changed files with 72 additions and 19 deletions
@ -1,26 +1,16 @@ |
|||
<script setup> |
|||
import DigitalClock from "./components/DigitalClock.vue" |
|||
</script> |
|||
|
|||
<template> |
|||
<img alt="Vue logo" src="./assets/logo.png"> |
|||
<HelloWorld msg="Welcome to Your Vue.js App"/> |
|||
<DigitalClock /> |
|||
</template> |
|||
|
|||
<script> |
|||
import HelloWorld from './components/HelloWorld.vue' |
|||
|
|||
export default { |
|||
name: 'App', |
|||
components: { |
|||
HelloWorld |
|||
} |
|||
} |
|||
</script> |
|||
|
|||
<style> |
|||
|
|||
#app { |
|||
font-family: Avenir, Helvetica, Arial, sans-serif; |
|||
-webkit-font-smoothing: antialiased; |
|||
-moz-osx-font-smoothing: grayscale; |
|||
text-align: center; |
|||
color: #2c3e50; |
|||
margin-top: 60px; |
|||
} |
|||
|
|||
</style> |
@ -0,0 +1,63 @@ |
|||
<script> |
|||
export default { |
|||
name: "DigitalClock", |
|||
data() { |
|||
return { |
|||
hours: 0, |
|||
minutes: 0, |
|||
seconds: 0 |
|||
} |
|||
}, |
|||
mounted() { |
|||
setInterval(() => this.setTime(), 1000) |
|||
}, |
|||
methods: { |
|||
setTime() { |
|||
const date = new Date(); |
|||
let hours = date.getHours(); |
|||
let minutes = date.getMinutes(); |
|||
let seconds = date.getSeconds(); |
|||
hours = hours <= 9 ? `${hours}`.padStart(2, 0) : hours; |
|||
minutes = minutes <= 9 ? `${minutes}`.padStart(2, 0) : minutes; |
|||
seconds = seconds <= 9 ? `${seconds}`.padStart(2, 0) : seconds; |
|||
this.hours = hours; |
|||
this.minutes = minutes; |
|||
this.seconds = seconds; |
|||
} |
|||
} |
|||
} |
|||
</script> |
|||
|
|||
<template> |
|||
<h1>Digital Clock</h1> |
|||
<div class="container"> |
|||
<div class="LCD"> |
|||
<div class="hours"><p>{{ hours }}</p></div> |
|||
<div class="divider"><p>:</p></div> |
|||
<div class="minutes"><p>{{ minutes }}</p></div> |
|||
<div class="divider"><p>:</p></div> |
|||
<div class="seconds"><p>{{ seconds }}</p></div> |
|||
</div> |
|||
</div> |
|||
</template> |
|||
|
|||
<style scoped> |
|||
|
|||
.LCD { |
|||
display:inline-flex; |
|||
color: red; |
|||
background: black; |
|||
width: 360px; |
|||
height: 315px; |
|||
|
|||
|
|||
} |
|||
.LCD > div { |
|||
font-family: "alarm clock"; |
|||
font-size: 100px; |
|||
|
|||
} |
|||
p{ |
|||
text-align: center; |
|||
} |
|||
</style> |
Loading…
Reference in new issue