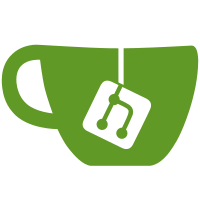
21 changed files with 471 additions and 0 deletions
@ -0,0 +1,5 @@ |
|||
.pio |
|||
.vscode/.browse.c_cpp.db* |
|||
.vscode/c_cpp_properties.json |
|||
.vscode/launch.json |
|||
.vscode/ipch |
@ -0,0 +1,10 @@ |
|||
{ |
|||
// See http://go.microsoft.com/fwlink/?LinkId=827846 |
|||
// for the documentation about the extensions.json format |
|||
"recommendations": [ |
|||
"platformio.platformio-ide" |
|||
], |
|||
"unwantedRecommendations": [ |
|||
"ms-vscode.cpptools-extension-pack" |
|||
] |
|||
} |
@ -0,0 +1,16 @@ |
|||
<!DOCTYPE html> |
|||
<html> |
|||
<head> |
|||
<title>ESP32 Web Server</title> |
|||
<meta name="viewport" content="width=device-width, initial-scale=1"> |
|||
<link rel="icon" href="data:,"> |
|||
<link rel="stylesheet" type="text/css" href="style.css"> |
|||
</head> |
|||
<body> |
|||
<h1>ESP32 Web Server</h1> |
|||
<p>GPIO state: <strong> %STATE%</strong></p> |
|||
<p><a href="/on"><button class="button">ON</button></a></p> |
|||
<p><a href="/off"><button class="button button2">OFF</button></a></p> |
|||
</body> |
|||
</html> |
|||
|
@ -0,0 +1,28 @@ |
|||
html { |
|||
font-family: Helvetica; |
|||
display: inline-block; |
|||
margin: 0px auto; |
|||
text-align: center; |
|||
} |
|||
h1{ |
|||
color: #0F3376; |
|||
padding: 2vh; |
|||
} |
|||
p{ |
|||
font-size: 1.5rem; |
|||
} |
|||
.button { |
|||
display: inline-block; |
|||
background-color: #008CBA; |
|||
border: none; |
|||
border-radius: 4px; |
|||
color: white; |
|||
padding: 16px 40px; |
|||
text-decoration: none; |
|||
font-size: 30px; |
|||
margin: 2px; |
|||
cursor: pointer; |
|||
} |
|||
.button2 { |
|||
background-color: #f44336; |
|||
} |
@ -0,0 +1,16 @@ |
|||
; PlatformIO Project Configuration File |
|||
; |
|||
; Build options: build flags, source filter |
|||
; Upload options: custom upload port, speed and extra flags |
|||
; Library options: dependencies, extra library storages |
|||
; Advanced options: extra scripting |
|||
; |
|||
; Please visit documentation for the other options and examples |
|||
; https://docs.platformio.org/page/projectconf.html |
|||
|
|||
[env:esp32dev] |
|||
platform = espressif32 |
|||
board = esp32dev |
|||
framework = arduino |
|||
lib_deps = me-no-dev/ESPAsyncWebServer@^3.6.0 |
|||
monitor_speed=115200 |
@ -0,0 +1,88 @@ |
|||
/*********
|
|||
Rui Santos |
|||
Complete project details at https://randomnerdtutorials.com
|
|||
*********/ |
|||
|
|||
// Import required libraries
|
|||
#include "WiFi.h" |
|||
#include "ESPAsyncWebServer.h" |
|||
#include "SPIFFS.h" |
|||
|
|||
// Replace with your network credentials
|
|||
const char* ssid = "tksteti"; |
|||
const char* password = "ProsimTeNevim"; |
|||
|
|||
// Set LED GPIO
|
|||
const int ledPin = 2; |
|||
// Stores LED state
|
|||
String ledState; |
|||
|
|||
// Create AsyncWebServer object on port 80
|
|||
AsyncWebServer server(80); |
|||
|
|||
// Replaces placeholder with LED state value
|
|||
String processor(const String& var){ |
|||
Serial.println(var); |
|||
if(var == "STATE"){ |
|||
if(digitalRead(ledPin)){ |
|||
ledState = "ON"; |
|||
} |
|||
else{ |
|||
ledState = "OFF"; |
|||
} |
|||
Serial.print(ledState); |
|||
return ledState; |
|||
} |
|||
return String(); |
|||
} |
|||
|
|||
void setup(){ |
|||
// Serial port for debugging purposes
|
|||
Serial.begin(115200); |
|||
pinMode(ledPin, OUTPUT); |
|||
|
|||
// Initialize SPIFFS
|
|||
if(!SPIFFS.begin(true)){ |
|||
Serial.println("An Error has occurred while mounting SPIFFS"); |
|||
return; |
|||
} |
|||
|
|||
// Connect to Wi-Fi
|
|||
WiFi.begin(ssid, password); |
|||
while (WiFi.status() != WL_CONNECTED) { |
|||
delay(1000); |
|||
Serial.println("Connecting to WiFi.."); |
|||
} |
|||
|
|||
// Print ESP32 Local IP Address
|
|||
Serial.println(WiFi.localIP()); |
|||
|
|||
// Route for root / web page
|
|||
server.on("/", HTTP_GET, [](AsyncWebServerRequest *request){ |
|||
request->send(SPIFFS, "/index.html", String(), false, processor); |
|||
}); |
|||
|
|||
// Route to load style.css file
|
|||
server.on("/style.css", HTTP_GET, [](AsyncWebServerRequest *request){ |
|||
request->send(SPIFFS, "/style.css", "text/css"); |
|||
}); |
|||
|
|||
// Route to set GPIO to HIGH
|
|||
server.on("/on", HTTP_GET, [](AsyncWebServerRequest *request){ |
|||
digitalWrite(ledPin, HIGH); |
|||
request->send(SPIFFS, "/index.html", String(), false, processor); |
|||
}); |
|||
|
|||
// Route to set GPIO to LOW
|
|||
server.on("/off", HTTP_GET, [](AsyncWebServerRequest *request){ |
|||
digitalWrite(ledPin, LOW); |
|||
request->send(SPIFFS, "/index.html", String(), false, processor); |
|||
}); |
|||
|
|||
// Start server
|
|||
server.begin(); |
|||
} |
|||
|
|||
void loop(){ |
|||
|
|||
} |
@ -0,0 +1,11 @@ |
|||
|
|||
This directory is intended for PlatformIO Test Runner and project tests. |
|||
|
|||
Unit Testing is a software testing method by which individual units of |
|||
source code, sets of one or more MCU program modules together with associated |
|||
control data, usage procedures, and operating procedures, are tested to |
|||
determine whether they are fit for use. Unit testing finds problems early |
|||
in the development cycle. |
|||
|
|||
More information about PlatformIO Unit Testing: |
|||
- https://docs.platformio.org/en/latest/advanced/unit-testing/index.html |
@ -0,0 +1,5 @@ |
|||
.pio |
|||
.vscode/.browse.c_cpp.db* |
|||
.vscode/c_cpp_properties.json |
|||
.vscode/launch.json |
|||
.vscode/ipch |
@ -0,0 +1,10 @@ |
|||
{ |
|||
// See http://go.microsoft.com/fwlink/?LinkId=827846 |
|||
// for the documentation about the extensions.json format |
|||
"recommendations": [ |
|||
"platformio.platformio-ide" |
|||
], |
|||
"unwantedRecommendations": [ |
|||
"ms-vscode.cpptools-extension-pack" |
|||
] |
|||
} |
@ -0,0 +1 @@ |
|||
Hello World! from text.txt |
@ -0,0 +1,16 @@ |
|||
; PlatformIO Project Configuration File |
|||
; |
|||
; Build options: build flags, source filter |
|||
; Upload options: custom upload port, speed and extra flags |
|||
; Library options: dependencies, extra library storages |
|||
; Advanced options: extra scripting |
|||
; |
|||
; Please visit documentation for the other options and examples |
|||
; https://docs.platformio.org/page/projectconf.html |
|||
|
|||
[env:esp32dev] |
|||
platform = espressif32 |
|||
board = esp32dev |
|||
framework = arduino |
|||
monitor_speed = 115200 |
|||
board_build.filesystem = littlefs |
@ -0,0 +1,32 @@ |
|||
/*********
|
|||
Rui Santos & Sara Santos - Random Nerd Tutorials |
|||
Complete project details at https://RandomNerdTutorials.com/esp32-vs-code-platformio-littlefs/
|
|||
*********/ |
|||
|
|||
#include <Arduino.h> |
|||
#include "LittleFS.h" |
|||
|
|||
void setup() { |
|||
Serial.begin(115200); |
|||
|
|||
if(!LittleFS.begin(true)){ |
|||
Serial.println("An Error has occurred while mounting LittleFS"); |
|||
return; |
|||
} |
|||
|
|||
File file = LittleFS.open("/text.txt"); |
|||
if(!file){ |
|||
Serial.println("Failed to open file for reading"); |
|||
return; |
|||
} |
|||
|
|||
Serial.println("File Content:"); |
|||
while(file.available()){ |
|||
Serial.write(file.read()); |
|||
} |
|||
file.close(); |
|||
} |
|||
|
|||
void loop() { |
|||
|
|||
} |
@ -0,0 +1,11 @@ |
|||
|
|||
This directory is intended for PlatformIO Test Runner and project tests. |
|||
|
|||
Unit Testing is a software testing method by which individual units of |
|||
source code, sets of one or more MCU program modules together with associated |
|||
control data, usage procedures, and operating procedures, are tested to |
|||
determine whether they are fit for use. Unit testing finds problems early |
|||
in the development cycle. |
|||
|
|||
More information about PlatformIO Unit Testing: |
|||
- https://docs.platformio.org/en/latest/advanced/unit-testing/index.html |
@ -0,0 +1,5 @@ |
|||
.pio |
|||
.vscode/.browse.c_cpp.db* |
|||
.vscode/c_cpp_properties.json |
|||
.vscode/launch.json |
|||
.vscode/ipch |
@ -0,0 +1,10 @@ |
|||
{ |
|||
// See http://go.microsoft.com/fwlink/?LinkId=827846 |
|||
// for the documentation about the extensions.json format |
|||
"recommendations": [ |
|||
"platformio.platformio-ide" |
|||
], |
|||
"unwantedRecommendations": [ |
|||
"ms-vscode.cpptools-extension-pack" |
|||
] |
|||
} |
@ -0,0 +1,17 @@ |
|||
; PlatformIO Project Configuration File |
|||
; |
|||
; Build options: build flags, source filter |
|||
; Upload options: custom upload port, speed and extra flags |
|||
; Library options: dependencies, extra library storages |
|||
; Advanced options: extra scripting |
|||
; |
|||
; Please visit documentation for the other options and examples |
|||
; https://docs.platformio.org/page/projectconf.html |
|||
|
|||
[env:esp32dev] |
|||
platform = espressif32 |
|||
board = esp32dev |
|||
framework = arduino |
|||
monitor_speed = 115200 |
|||
lib_deps = me-no-dev/ESPAsyncWebServer@^3.6.0 |
|||
board_build.filesystem = littlefs |
@ -0,0 +1,179 @@ |
|||
/*********
|
|||
Rui Santos & Sara Santos - Random Nerd Tutorials |
|||
Complete project details at https://RandomNerdTutorials.com/esp32-esp8266-input-data-html-form/
|
|||
Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files. |
|||
The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software. |
|||
*********/ |
|||
#include <Arduino.h> |
|||
#ifdef ESP32 |
|||
#include <WiFi.h> |
|||
#include <AsyncTCP.h> |
|||
#include <LittleFS.h> |
|||
#else |
|||
#include <ESP8266WiFi.h> |
|||
#include <ESPAsyncTCP.h> |
|||
#include <Hash.h> |
|||
#include <LittleFS.h> |
|||
#endif |
|||
#include <ESPAsyncWebServer.h> |
|||
|
|||
AsyncWebServer server(80); |
|||
|
|||
// REPLACE WITH YOUR NETWORK CREDENTIALS
|
|||
const char* ssid = "tksteti"; |
|||
const char* password = "ProsimTeNevim"; |
|||
|
|||
const char* PARAM_STRING = "inputString"; |
|||
const char* PARAM_INT = "inputInt"; |
|||
const char* PARAM_FLOAT = "inputFloat"; |
|||
|
|||
// HTML web page to handle 3 input fields (inputString, inputInt, inputFloat)
|
|||
const char index_html[] PROGMEM = R"rawliteral( |
|||
<!DOCTYPE HTML><html><head> |
|||
<title>ESP Input Form</title> |
|||
<meta name="viewport" content="width=device-width, initial-scale=1"> |
|||
<script> |
|||
function submitMessage() { |
|||
alert("Saved value to ESP LittleFS"); |
|||
setTimeout(function(){ document.location.reload(false); }, 500); |
|||
} |
|||
</script></head><body> |
|||
<form action="/get" target="hidden-form"> |
|||
inputString (current value %inputString%): <input type="text" name="inputString"> |
|||
<input type="submit" value="Submit" onclick="submitMessage()"> |
|||
</form><br> |
|||
<form action="/get" target="hidden-form"> |
|||
inputInt (current value %inputInt%): <input type="number " name="inputInt"> |
|||
<input type="submit" value="Submit" onclick="submitMessage()"> |
|||
</form><br> |
|||
<form action="/get" target="hidden-form"> |
|||
inputFloat (current value %inputFloat%): <input type="number " name="inputFloat"> |
|||
<input type="submit" value="Submit" onclick="submitMessage()"> |
|||
</form> |
|||
<iframe style="display:none" name="hidden-form"></iframe> |
|||
</body></html>)rawliteral"; |
|||
|
|||
void notFound(AsyncWebServerRequest *request) { |
|||
request->send(404, "text/plain", "Not found"); |
|||
} |
|||
|
|||
String readFile(fs::FS &fs, const char * path){ |
|||
Serial.printf("Reading file: %s\r\n", path); |
|||
File file = fs.open(path, "r"); |
|||
if(!file || file.isDirectory()){ |
|||
Serial.println("- empty file or failed to open file"); |
|||
return String(); |
|||
} |
|||
Serial.println("- read from file:"); |
|||
String fileContent; |
|||
while(file.available()){ |
|||
fileContent+=String((char)file.read()); |
|||
} |
|||
file.close(); |
|||
Serial.println(fileContent); |
|||
return fileContent; |
|||
} |
|||
|
|||
void writeFile(fs::FS &fs, const char * path, const char * message){ |
|||
Serial.printf("Writing file: %s\r\n", path); |
|||
File file = fs.open(path, "w"); |
|||
if(!file){ |
|||
Serial.println("- failed to open file for writing"); |
|||
return; |
|||
} |
|||
if(file.print(message)){ |
|||
Serial.println("- file written"); |
|||
} else { |
|||
Serial.println("- write failed"); |
|||
} |
|||
file.close(); |
|||
} |
|||
|
|||
// Replaces placeholder with stored values
|
|||
String processor(const String& var){ |
|||
//Serial.println(var);
|
|||
if(var == "inputString"){ |
|||
return readFile(LittleFS, "/inputString.txt"); |
|||
} |
|||
else if(var == "inputInt"){ |
|||
return readFile(LittleFS, "/inputInt.txt"); |
|||
} |
|||
else if(var == "inputFloat"){ |
|||
return readFile(LittleFS, "/inputFloat.txt"); |
|||
} |
|||
return String(); |
|||
} |
|||
|
|||
void setup() { |
|||
Serial.begin(115200); |
|||
// Initialize LittleFS
|
|||
#ifdef ESP32 |
|||
if(!LittleFS.begin(true)){ |
|||
Serial.println("An Error has occurred while mounting LittleFS"); |
|||
return; |
|||
} |
|||
#else |
|||
if(!LittleFS.begin()){ |
|||
Serial.println("An Error has occurred while mounting LittleFS"); |
|||
return; |
|||
} |
|||
#endif |
|||
|
|||
WiFi.mode(WIFI_STA); |
|||
WiFi.begin(ssid, password); |
|||
if (WiFi.waitForConnectResult() != WL_CONNECTED) { |
|||
Serial.println("WiFi Failed!"); |
|||
return; |
|||
} |
|||
Serial.println(); |
|||
Serial.print("IP Address: "); |
|||
Serial.println(WiFi.localIP()); |
|||
|
|||
// Send web page with input fields to client
|
|||
server.on("/", HTTP_GET, [](AsyncWebServerRequest *request){ |
|||
request->send_P(200, "text/html", index_html, processor); |
|||
}); |
|||
|
|||
// Send a GET request to <ESP_IP>/get?inputString=<inputMessage>
|
|||
server.on("/get", HTTP_GET, [] (AsyncWebServerRequest *request) { |
|||
String inputMessage; |
|||
// GET inputString value on <ESP_IP>/get?inputString=<inputMessage>
|
|||
if (request->hasParam(PARAM_STRING)) { |
|||
inputMessage = request->getParam(PARAM_STRING)->value(); |
|||
writeFile(LittleFS, "/inputString.txt", inputMessage.c_str()); |
|||
} |
|||
// GET inputInt value on <ESP_IP>/get?inputInt=<inputMessage>
|
|||
else if (request->hasParam(PARAM_INT)) { |
|||
inputMessage = request->getParam(PARAM_INT)->value(); |
|||
writeFile(LittleFS, "/inputInt.txt", inputMessage.c_str()); |
|||
} |
|||
// GET inputFloat value on <ESP_IP>/get?inputFloat=<inputMessage>
|
|||
else if (request->hasParam(PARAM_FLOAT)) { |
|||
inputMessage = request->getParam(PARAM_FLOAT)->value(); |
|||
writeFile(LittleFS, "/inputFloat.txt", inputMessage.c_str()); |
|||
} |
|||
else { |
|||
inputMessage = "No message sent"; |
|||
} |
|||
Serial.println(inputMessage); |
|||
request->send(200, "text/text", inputMessage); |
|||
}); |
|||
server.onNotFound(notFound); |
|||
server.begin(); |
|||
} |
|||
|
|||
void loop() { |
|||
// To access your stored values on inputString, inputInt, inputFloat
|
|||
String yourInputString = readFile(LittleFS, "/inputString.txt"); |
|||
Serial.print("*** Your inputString: "); |
|||
Serial.println(yourInputString); |
|||
|
|||
int yourInputInt = readFile(LittleFS, "/inputInt.txt").toInt(); |
|||
Serial.print("*** Your inputInt: "); |
|||
Serial.println(yourInputInt); |
|||
|
|||
float yourInputFloat = readFile(LittleFS, "/inputFloat.txt").toFloat(); |
|||
Serial.print("*** Your inputFloat: "); |
|||
Serial.println(yourInputFloat); |
|||
delay(5000); |
|||
} |
@ -0,0 +1,11 @@ |
|||
|
|||
This directory is intended for PlatformIO Test Runner and project tests. |
|||
|
|||
Unit Testing is a software testing method by which individual units of |
|||
source code, sets of one or more MCU program modules together with associated |
|||
control data, usage procedures, and operating procedures, are tested to |
|||
determine whether they are fit for use. Unit testing finds problems early |
|||
in the development cycle. |
|||
|
|||
More information about PlatformIO Unit Testing: |
|||
- https://docs.platformio.org/en/latest/advanced/unit-testing/index.html |
Loading…
Reference in new issue