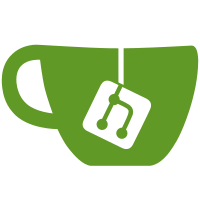
13 changed files with 389 additions and 0 deletions
@ -0,0 +1,22 @@ |
|||||
|
from django.db import models |
||||
|
|
||||
|
from modelcluster.fields import ParentalKey |
||||
|
|
||||
|
from wagtail.core.models import Page, Orderable |
||||
|
from wagtail.core.fields import RichTextField |
||||
|
from wagtail.images.edit_handlers import ImageChooserPanel |
||||
|
from wagtail.admin.edit_handlers import FieldPanel, InlinePanel |
||||
|
|
||||
|
|
||||
|
class HomePage(Page): |
||||
|
body = RichTextField(blank=True) |
||||
|
address = RichTextField(blank=True) |
||||
|
image = models.ForeignKey( |
||||
|
'wagtailimages.Image', on_delete=models.CASCADE, related_name='+' |
||||
|
) |
||||
|
|
||||
|
content_panels = Page.content_panels + [ |
||||
|
FieldPanel('body', classname="full"), |
||||
|
FieldPanel('address', classname="full"), |
||||
|
ImageChooserPanel('image'), |
||||
|
] |
@ -0,0 +1,53 @@ |
|||||
|
from django.db import models |
||||
|
|
||||
|
from modelcluster.fields import ParentalKey |
||||
|
|
||||
|
from wagtail.core.models import Page, Orderable |
||||
|
from wagtail.core.fields import RichTextField |
||||
|
from wagtail.admin.edit_handlers import FieldPanel, InlinePanel |
||||
|
from wagtail.images.edit_handlers import ImageChooserPanel |
||||
|
from wagtail.search import index |
||||
|
|
||||
|
|
||||
|
class ProgramList(Page): |
||||
|
year = models.PositiveIntegerField(blank=True) |
||||
|
intro = RichTextField(blank=True) |
||||
|
image = models.ForeignKey( |
||||
|
'wagtailimages.Image', on_delete=models.CASCADE, related_name='+' |
||||
|
) |
||||
|
|
||||
|
parent_page_types = ['home.HomePage', ] |
||||
|
|
||||
|
content_panels = Page.content_panels + [ |
||||
|
FieldPanel('year'), |
||||
|
FieldPanel('intro', classname="full"), |
||||
|
ImageChooserPanel('image'), |
||||
|
] |
||||
|
|
||||
|
|
||||
|
class ProgramEntry(Page): |
||||
|
date = models.DateTimeField("Datum a čas projekce") |
||||
|
place = models.CharField(max_length=64) |
||||
|
release_date = models.DateField("Datum vydání filmu") |
||||
|
director = models.CharField(max_length=64) |
||||
|
country = models.CharField(max_length=64) |
||||
|
annotation = RichTextField(blank=True) |
||||
|
image = models.ForeignKey( |
||||
|
'wagtailimages.Image', on_delete=models.CASCADE, related_name='+' |
||||
|
) |
||||
|
|
||||
|
parent_page_types = ['program.ProgramList', ] |
||||
|
|
||||
|
search_fields = Page.search_fields + [ |
||||
|
index.SearchField('annotation'), |
||||
|
] |
||||
|
|
||||
|
content_panels = Page.content_panels + [ |
||||
|
FieldPanel('date'), |
||||
|
FieldPanel('place'), |
||||
|
FieldPanel('release_date'), |
||||
|
FieldPanel('director'), |
||||
|
FieldPanel('country'), |
||||
|
FieldPanel('annotation', classname="full"), |
||||
|
ImageChooserPanel('image'), |
||||
|
] |
@ -0,0 +1,25 @@ |
|||||
|
# Filmový festival |
||||
|
|
||||
|
Webové stránky filmového festivalu zpravidla obsahují: |
||||
|
|
||||
|
- domovskou stránku s průvodním textem a hlavním "bannerem/obrázkem" festivalu |
||||
|
- statické stránky s informacemi o festivalu, pořadatelích nebo sponzorech |
||||
|
- program |
||||
|
|
||||
|
## homepage |
||||
|
- hlavní foto |
||||
|
- průvodní text |
||||
|
|
||||
|
## statické stránky |
||||
|
- formátovaný text |
||||
|
- obrázky |
||||
|
|
||||
|
## program |
||||
|
- datum a čas projekce |
||||
|
- místo projekce |
||||
|
- název filmu |
||||
|
- datum vydání filmu |
||||
|
- režie |
||||
|
- země |
||||
|
- anotace k filmu |
||||
|
- obrázek k filmu |
@ -0,0 +1,35 @@ |
|||||
|
from django.db import models |
||||
|
|
||||
|
from modelcluster.fields import ParentalKey |
||||
|
|
||||
|
from wagtail.core.models import Page, Orderable |
||||
|
from wagtail.core.fields import RichTextField |
||||
|
from wagtail.admin.edit_handlers import FieldPanel, InlinePanel |
||||
|
from wagtail.images.edit_handlers import ImageChooserPanel |
||||
|
from wagtail.search import index |
||||
|
|
||||
|
|
||||
|
class StrankaPage(Page): |
||||
|
body = RichTextField(blank=True) |
||||
|
|
||||
|
search_fields = Page.search_fields + [ |
||||
|
index.SearchField('body'), |
||||
|
] |
||||
|
|
||||
|
content_panels = Page.content_panels + [ |
||||
|
FieldPanel('body', classname="full"), |
||||
|
InlinePanel('gallery_images', label="Obrázky"), |
||||
|
] |
||||
|
|
||||
|
|
||||
|
class StrankaPageGalleryImage(Orderable): |
||||
|
page = ParentalKey(StrankaPage, on_delete=models.CASCADE, related_name='gallery_images') |
||||
|
image = models.ForeignKey( |
||||
|
'wagtailimages.Image', on_delete=models.CASCADE, related_name='+' |
||||
|
) |
||||
|
caption = models.CharField(blank=True, max_length=250) |
||||
|
|
||||
|
panels = [ |
||||
|
ImageChooserPanel('image'), |
||||
|
FieldPanel('caption'), |
||||
|
] |
@ -0,0 +1,61 @@ |
|||||
|
from django.db import models |
||||
|
|
||||
|
from modelcluster.fields import ParentalKey |
||||
|
|
||||
|
from wagtail.core.models import Page, Orderable |
||||
|
from wagtail.core.fields import RichTextField |
||||
|
from wagtail.admin.edit_handlers import FieldPanel, InlinePanel |
||||
|
from wagtail.images.edit_handlers import ImageChooserPanel |
||||
|
from wagtail.search import index |
||||
|
|
||||
|
|
||||
|
class CategoryList(Page): |
||||
|
intro = RichTextField(blank=True) |
||||
|
image = models.ForeignKey( |
||||
|
'wagtailimages.Image', on_delete=models.CASCADE, related_name='+' |
||||
|
) |
||||
|
|
||||
|
parent_page_types = ['home.HomePage', ] |
||||
|
|
||||
|
content_panels = Page.content_panels + [ |
||||
|
FieldPanel('intro', classname="full"), |
||||
|
ImageChooserPanel('image'), |
||||
|
] |
||||
|
|
||||
|
|
||||
|
class Product(Page): |
||||
|
price = models.PositiveIntegerField() |
||||
|
description = RichTextField(blank=True) |
||||
|
|
||||
|
def main_image(self): |
||||
|
gallery_item = self.gallery_images.first() |
||||
|
if gallery_item: |
||||
|
return gallery_item.image |
||||
|
else: |
||||
|
return None |
||||
|
|
||||
|
parent_page_types = ['catalog.CategoryList', ] |
||||
|
|
||||
|
search_fields = Page.search_fields + [ |
||||
|
index.SearchField('description'), |
||||
|
] |
||||
|
|
||||
|
content_panels = Page.content_panels + [ |
||||
|
FieldPanel('price'), |
||||
|
FieldPanel('description', classname="full"), |
||||
|
InlinePanel('gallery_images', label="Obrázky"), |
||||
|
] |
||||
|
|
||||
|
|
||||
|
|
||||
|
class ProductImage(Orderable): |
||||
|
page = ParentalKey(BlogPage, on_delete=models.CASCADE, related_name='product_images') |
||||
|
image = models.ForeignKey( |
||||
|
'wagtailimages.Image', on_delete=models.CASCADE, related_name='+' |
||||
|
) |
||||
|
caption = models.CharField(blank=True, max_length=250) |
||||
|
|
||||
|
panels = [ |
||||
|
ImageChooserPanel('image'), |
||||
|
FieldPanel('caption'), |
||||
|
] |
@ -0,0 +1,19 @@ |
|||||
|
# Showroom |
||||
|
|
||||
|
Webové stránky "showroom" zpravidla obsahují: |
||||
|
|
||||
|
- domovskou stránku s hlavním produktem (scoll page design) |
||||
|
- další stránky s informacemi o produktu (např. technické specifikace) |
||||
|
- katalog dalších produktů |
||||
|
|
||||
|
## statické stránky |
||||
|
- formátovaný text |
||||
|
- obrázky |
||||
|
|
||||
|
## katalog |
||||
|
- obrázky |
||||
|
- název |
||||
|
- popis |
||||
|
- kategorie produktu |
||||
|
- cena |
||||
|
- parametry (dle produktu) |
@ -0,0 +1,35 @@ |
|||||
|
from django.db import models |
||||
|
|
||||
|
from modelcluster.fields import ParentalKey |
||||
|
|
||||
|
from wagtail.core.models import Page, Orderable |
||||
|
from wagtail.core.fields import RichTextField |
||||
|
from wagtail.admin.edit_handlers import FieldPanel, InlinePanel |
||||
|
from wagtail.images.edit_handlers import ImageChooserPanel |
||||
|
from wagtail.search import index |
||||
|
|
||||
|
|
||||
|
class StrankaPage(Page): |
||||
|
body = RichTextField(blank=True) |
||||
|
|
||||
|
search_fields = Page.search_fields + [ |
||||
|
index.SearchField('body'), |
||||
|
] |
||||
|
|
||||
|
content_panels = Page.content_panels + [ |
||||
|
FieldPanel('body', classname="full"), |
||||
|
InlinePanel('gallery_images', label="Obrázky"), |
||||
|
] |
||||
|
|
||||
|
|
||||
|
class StrankaPageGalleryImage(Orderable): |
||||
|
page = ParentalKey(StrankaPage, on_delete=models.CASCADE, related_name='gallery_images') |
||||
|
image = models.ForeignKey( |
||||
|
'wagtailimages.Image', on_delete=models.CASCADE, related_name='+' |
||||
|
) |
||||
|
caption = models.CharField(blank=True, max_length=250) |
||||
|
|
||||
|
panels = [ |
||||
|
ImageChooserPanel('image'), |
||||
|
FieldPanel('caption'), |
||||
|
] |
@ -0,0 +1,55 @@ |
|||||
|
from django.db import models |
||||
|
|
||||
|
from modelcluster.fields import ParentalKey |
||||
|
|
||||
|
from wagtail.core.models import Page, Orderable |
||||
|
from wagtail.core.fields import RichTextField |
||||
|
from wagtail.admin.edit_handlers import FieldPanel, InlinePanel |
||||
|
from wagtail.images.edit_handlers import ImageChooserPanel |
||||
|
from wagtail.search import index |
||||
|
|
||||
|
|
||||
|
class BlogIndexPage(Page): |
||||
|
intro = RichTextField(blank=True) |
||||
|
|
||||
|
content_panels = Page.content_panels + [ |
||||
|
FieldPanel('intro', classname="full") |
||||
|
] |
||||
|
|
||||
|
|
||||
|
class BlogPage(Page): |
||||
|
date = models.DateField("Post date") |
||||
|
intro = models.CharField(max_length=250) |
||||
|
body = RichTextField(blank=True) |
||||
|
|
||||
|
def main_image(self): |
||||
|
gallery_item = self.gallery_images.first() |
||||
|
if gallery_item: |
||||
|
return gallery_item.image |
||||
|
else: |
||||
|
return None |
||||
|
|
||||
|
search_fields = Page.search_fields + [ |
||||
|
index.SearchField('intro'), |
||||
|
index.SearchField('body'), |
||||
|
] |
||||
|
|
||||
|
content_panels = Page.content_panels + [ |
||||
|
FieldPanel('date'), |
||||
|
FieldPanel('intro'), |
||||
|
FieldPanel('body', classname="full"), |
||||
|
InlinePanel('gallery_images', label="Obrázky"), |
||||
|
] |
||||
|
|
||||
|
|
||||
|
class BlogPageGalleryImage(Orderable): |
||||
|
page = ParentalKey(BlogPage, on_delete=models.CASCADE, related_name='gallery_images') |
||||
|
image = models.ForeignKey( |
||||
|
'wagtailimages.Image', on_delete=models.CASCADE, related_name='+' |
||||
|
) |
||||
|
caption = models.CharField(blank=True, max_length=250) |
||||
|
|
||||
|
panels = [ |
||||
|
ImageChooserPanel('image'), |
||||
|
FieldPanel('caption'), |
||||
|
] |
@ -0,0 +1,22 @@ |
|||||
|
from django.db import models |
||||
|
|
||||
|
from modelcluster.fields import ParentalKey |
||||
|
|
||||
|
from wagtail.core.models import Page, Orderable |
||||
|
from wagtail.core.fields import RichTextField |
||||
|
from wagtail.images.edit_handlers import ImageChooserPanel |
||||
|
from wagtail.admin.edit_handlers import FieldPanel, InlinePanel |
||||
|
|
||||
|
|
||||
|
class HomePage(Page): |
||||
|
body = RichTextField(blank=True) |
||||
|
address = RichTextField(blank=True) |
||||
|
image = models.ForeignKey( |
||||
|
'wagtailimages.Image', on_delete=models.CASCADE, related_name='+' |
||||
|
) |
||||
|
|
||||
|
content_panels = Page.content_panels + [ |
||||
|
FieldPanel('body', classname="full"), |
||||
|
FieldPanel('address', classname="full"), |
||||
|
ImageChooserPanel('image'), |
||||
|
] |
@ -0,0 +1,27 @@ |
|||||
|
# Veterinární klinika |
||||
|
|
||||
|
Webové stránky veterinární kliniky zpravidla obsahují: |
||||
|
|
||||
|
- domovskou stránku s průvodním textem a hlavním "bannerem/obrázkem" |
||||
|
kontakt / adresu kliniky |
||||
|
- statické stránky s dalšími informacemi o klinice: vybavení, služby, popis pracoviště |
||||
|
- aktuality - informace provozu kliniky, nedostupnosti lékaře, atp. |
||||
|
- blog o činnosti kliniky (např. úspěšné léčby/příběhy pacientů) |
||||
|
|
||||
|
|
||||
|
## stránky |
||||
|
- formátovaný text |
||||
|
- obrázky |
||||
|
|
||||
|
## aktuality |
||||
|
- datum |
||||
|
- text zprávy |
||||
|
|
||||
|
## blog |
||||
|
- datum |
||||
|
- text zápisu |
||||
|
- obrázky |
||||
|
|
||||
|
## homepage |
||||
|
- adresa |
||||
|
- hlavní foto |
@ -0,0 +1,35 @@ |
|||||
|
from django.db import models |
||||
|
|
||||
|
from modelcluster.fields import ParentalKey |
||||
|
|
||||
|
from wagtail.core.models import Page, Orderable |
||||
|
from wagtail.core.fields import RichTextField |
||||
|
from wagtail.admin.edit_handlers import FieldPanel, InlinePanel |
||||
|
from wagtail.images.edit_handlers import ImageChooserPanel |
||||
|
from wagtail.search import index |
||||
|
|
||||
|
|
||||
|
class StrankaPage(Page): |
||||
|
body = RichTextField(blank=True) |
||||
|
|
||||
|
search_fields = Page.search_fields + [ |
||||
|
index.SearchField('body'), |
||||
|
] |
||||
|
|
||||
|
content_panels = Page.content_panels + [ |
||||
|
FieldPanel('body', classname="full"), |
||||
|
InlinePanel('gallery_images', label="Obrázky"), |
||||
|
] |
||||
|
|
||||
|
|
||||
|
class StrankaPageGalleryImage(Orderable): |
||||
|
page = ParentalKey(StrankaPage, on_delete=models.CASCADE, related_name='gallery_images') |
||||
|
image = models.ForeignKey( |
||||
|
'wagtailimages.Image', on_delete=models.CASCADE, related_name='+' |
||||
|
) |
||||
|
caption = models.CharField(blank=True, max_length=250) |
||||
|
|
||||
|
panels = [ |
||||
|
ImageChooserPanel('image'), |
||||
|
FieldPanel('caption'), |
||||
|
] |
Loading…
Reference in new issue